Create a Linux Service Monitoring Script
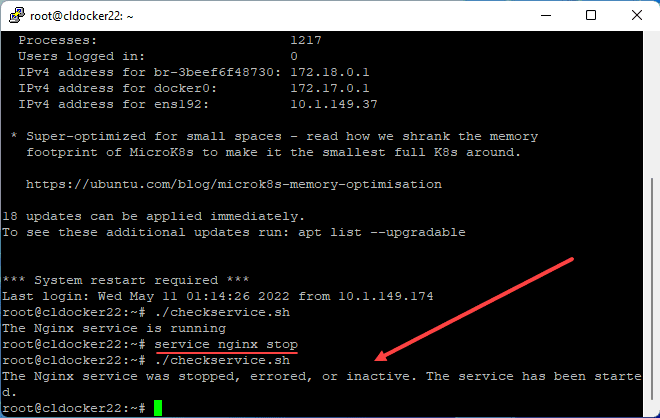
Highlights
- Txt file, we grep the contents of the fileThe grep command will look for the word “running”If it finds running we know the service is runningIf it doesn’t find the word running, it will move on to the “else” portion of the scriptIn the else logic, we run the service nginx start command to start the service if it isn’t runningWe can then echo contents out to pipe into a notification emailThe fi statement defines the end of the script blockAt the very bottom we are removing the output.
- Using a Bash script, we can easily create a piece of script code that can monitor a Linux service and then use CRON to run the script on a specified interval to monitor the status of a service.
- For the purposes of the demonstration of how to create a Linux service monitoring script, I will be monitoring Nginx to make sure the webserver is running.
You may have mission-critical workloads running on Linux Server such as Nginx that you want to monitor to ensure the service is healthy. Using a Bash script, we can easily create a piece of script code that can monitor a Linux service and then use CRON to run the script on a specified interval to monitor the status of a service. When I googled around for code snippets to create a Linux service monitoring script, I found several examples, but they didn’t work correctly when used with CRON. I want to show you guys how to create a Linux service monitoring script that works interactively and using CRON.
What is a Linux Bash script?
A Linux Bash script is a simple script that contains basic shell commands that you would type in at a Linux shell prompt. This makes it easy to get started with Linux Bash scripts. It is easy to throw commands into a shell script, just like you would interactively type into the shell prompt.
One of the great things about the Linux Bash script environment is it is built into Linux itself. There are no additional features you need to install to have the functionality. Also, you automatically have a task scheduler of sorts built into Linux to run the shell scripts with the Cron scheduler.
Create a Linux Service Monitoring Script
The process to create a Linux Service monitoring script is straightforward. You just need to have a code editor of sorts that can literally be notepad or some other text editor, and the sample code below. You can develop on the Linux server as well using nano, vi, or your editor of choice.
For the purposes of the demonstration of how to create a Linux service monitoring script, I will be monitoring Nginx to make sure the webserver is running. Let’s take a look at the script to do that.
Let’s walk through the script.
- At the top, we are defining some path variables
- The service nginx status command gets the status of the Nginx service
- We are outputting that to a file called output.txt
- Once we have created the output.txt file, we grep the contents of the file
- The grep command will look for the word “running”
- If it finds running we know the service is running
- If it doesn’t find the word running, it will move on to the “else” portion of the script
- In the else logic, we run the service nginx start command to start the service if it isn’t running
- We can then echo contents out to pipe into a notification email
- The fi statement defines the end of the script block
- At the very bottom we are removing the output.txt file to clean up after the script runs
SHELL=/bin/bash
PATH=/sbin:/bin:/usr/sbin:/usr/bin
#!/bin/bash
service nginx status > output.txt
if grep -q "running" output.txt; then
echo "The Nginx service is running"
else
service nginx start
echo "The Nginx service was stopped, errored, or inactive. The service has been started." | mail -s "The Nginx service was stopped" [email protected];
fi
sudo rm -f output.txt
Once you create a Linux monitoring script, you will need to save it. If you created it on a workstation outside of your Linux server, you will need to SCP the file locally to the Linux server to use. Using the example code above, I saved the script as checkservice.sh.
Additional tools needed?
Really, the only additional tools you may need to install involve the mail functionality. If you do not have the mailutils package installed, you will need to install that:
apt-get install mailutils
You may choose to use another local mail server. Just know you will need to fulfill the requirements of the specific mail server to call it when you create a Linux monitoring script.
Using CRON with Linux Bash Script
You may be wondering, how do we call the script once we have created it? You can run the script interactively if you want to being with. I prefer to do this to make sure the syntax and everything is working correctly.
If you can’t seem to execute your shell script, it may be because it is not set with the execute attribute. To make a shell script executable, you can do that with the following command:
chmod +x
Once you have made the script executable, you can execute it to test. With the Nginx service running, when we execute the script, we can see it properly detects the service is running.
Below, I am simulating the Nginx service being stopped or failed for whatever reason. As you can see now, when we run the checkservice.sh script, it detects the service is not running and issues the service start nginx command.
Create a Linux Service Monitoring Script Video
If you would like to watch a video tutorial of how to create a Linux service monitoring script, watch my video walkthrough below.
Create a Linux Service Monitoring Script FAQs
- What is a Bash script? A Linux Bash script is a simple human-readable script file that contains commands that you would type in manually at a shell prompt.
- How can you run a Bash script automatically? You can call Bash scripts from other Bash scripts. You can also schedule these to run using the Cron functionality found in Linux.
- What is Cron? Cron is the built-in scheduler found in Linux to run scripts. You can have Cron run Bash scripts automatically on a set interval and schedule.
- Why monitor Linux services? Business-critical Linux services need to be monitored to ensure they are running correctly. Using a Linux script to watch a service and ensure it is running is a great way to have self-healing infrastructure.
Wrapping Up
Linux Bash scripts are extremely powerful and provide a great way to perform Linux tasks in an automated way. Hopefully, this quick and easy tutorial will help anyone who wants to get started with Linux shell scripting to see how you can easily start reaping the benefits of running scripts in Linux as part of your system administration. The process to create a Linux service monitoring script is only an example of what you can do.
I would suggest using systemd for this, for example:
/bin/systemctl | grep nginx | grep running | awk '{print $4}'
To filter it’s status. Another syntax might work just fine. You can iterate through it so easily with a for loop.
A better way to define your bash environment would be:
#!/usr/bin/env bash
Making scripts more ‘env proof’