WSUS Import The Underlying Connection Was Closed New PowerShell Script
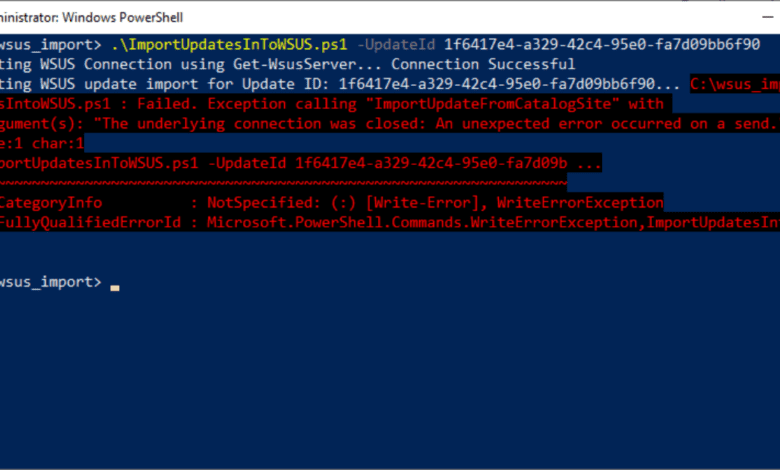
Microsoft recently announced that the WSUS import updates feature in the WSUS console would no longer be used. Instead, admins need to use a PowerShell script provided by Microsoft to import updates into WSUS. However, in my recent testing of the script on a Windows Server 2022 server with WSUS installed, after following the steps exactly from the Microsoft KB, I received the error, “The underlying connection was closed: An unexpected error occurred on a send.” Let’s look at the error and the fix for this issue.
If you want to get straight to the fix, skip in the contents to The Fix: Change SchUseStrongCrypto registry key.
Table of contents
- The error background
- Diagnosing the Issue
- The Fix: Change SchUseStrongCrypto registry key
- ServerSyncProxy Proxy and WSUS Console
- Points for consideration
- What is WSUS Server?
- The Role of TLS 1.2 and .NET Framework
- How Does WSUS Sync with Microsoft Update Catalog?
- What Might Cause an Unexpected Error in WSUS?
- Can Proxy Settings Impact WSUS Performance?
- Why Do WSUS Errors Often Involve Internet Explorer?
- What is the Role of .NET Framework in WSUS Operations?
- Why Does an Existing Connection Get Forcibly Closed?
- Can I Manually Import Updates in WSUS?
- Can WSUS Function Without Internet Explorer?
- Is There a Way to Access WSUS Server Through PowerShell?
- Can the WSUS Error Log Provide Insight into the Problem?
- Final Thoughts and Further Troubleshooting
The error background
The error “wsus import the underlying connection was closed” often presents as an “unexpected error” in the WSUS server. This error usually indicates an issue with the underlying connection between the WSUS server and the remote host or the server and the internet. In other cases, this could be a symptom of a transport connection problem.
I ran into the error using the new import method detailed here: WSUS and the Microsoft Update Catalog | Microsoft Learn.
Microsoft has now deprecated the old way to import updates using Internet Explorer and ActiveX controls. For many reasons, this method is no longer supported or works due to the old technologies involved.
The KB linked above has the PowerShell script here:
<#
.SYNOPSIS
Powershell script to import an update, or multiple updates into WSUS based on the UpdateID from the catalog.
.DESCRIPTION
This script takes user input and attempts to connect to the WSUS server.
Then it tries to import the update using the provided UpdateID from the catalog.
.INPUTS
The script takes WSUS server Name/IP, WSUS server port, SSL configuration option and UpdateID as input. UpdateID can be viewed and copied from the update details page for any update in the catalog, https://catalog.update.microsoft.com.
.OUTPUTS
Writes logging information to standard output.
.EXAMPLE
# Use with remote server IP, port and SSL
.ImportUpdateToWSUS.ps1 -WsusServer 127.0.0.1 -PortNumber 8531 -UseSsl -UpdateId 12345678-90ab-cdef-1234-567890abcdef
.EXAMPLE
# Use with remote server Name, port and SSL
.ImportUpdateToWSUS.ps1 -WsusServer WSUSServer1.us.contoso.com -PortNumber 8531 -UseSsl -UpdateId 12345678-90ab-cdef-1234-567890abcdef
.EXAMPLE
# Use with remote server IP, defaultport and no SSL
.ImportUpdateToWSUS.ps1 -WsusServer 127.0.0.1 -UpdateId 12345678-90ab-cdef-1234-567890abcdef
.EXAMPLE
# Use with localhost default port
.ImportUpdateToWSUS.ps1 -UpdateId 12345678-90ab-cdef-1234-567890abcdef
.EXAMPLE
# Use with localhost default port, file with updateID's
.ImportUpdateToWSUS.ps1 -UpdateIdFilePath .file.txt
.NOTES
# On error, try enabling TLS: https://learn.microsoft.com/mem/configmgr/core/plan-design/security/enable-tls-1-2-client
# Sample registry add for the WSUS server from command line. Restarts the WSUSService and IIS after adding:
reg add HKEY_LOCAL_MACHINESOFTWAREMicrosoft.NETFrameworkv4.0.30319 /V SchUseStrongCrypto /T REG_DWORD /D 1
## Sample registry add for the WSUS server from PowerShell. Restarts WSUSService and IIS after adding:
$registryPath = "HKLM:SoftwareMicrosoft.NETFrameworkv4.0.30319"
$Name = "SchUseStrongCrypto"
$value = "1"
if (!(Test-Path $registryPath)) {
New-Item -Path $registryPath -Force | Out-Null
}
New-ItemProperty -Path $registryPath -Name $name -Value $value -PropertyType DWORD -Force | Out-Null
Restart-Service WsusService, w3svc
# Update import logs/errors are under %ProgramFiles%Update ServicesLogFilesSoftwareDistribution.log
#>
param(
[Parameter(Mandatory = $false, HelpMessage = "Specifies the name of a WSUS server, if not specified connects to localhost")]
# Specifies the name of a WSUS server, if not specified connects to localhost.
[string]$WsusServer,
[Parameter(Mandatory = $false, HelpMessage = "Specifies the port number to use to communicate with the upstream WSUS server, default is 8530")]
# Specifies the port number to use to communicate with the upstream WSUS server, default is 8530.
[ValidateSet("80", "443", "8530", "8531")]
[int32]$PortNumber = 8530,
[Parameter(Mandatory = $false, HelpMessage = "Specifies that the WSUS server should use Secure Sockets Layer (SSL) via HTTPS to communicate with an upstream server")]
# Specifies that the WSUS server should use Secure Sockets Layer (SSL) via HTTPS to communicate with an upstream server.
[Switch]$UseSsl,
[Parameter(Mandatory = $true, HelpMessage = "Specifies the update Id we should import to WSUS", ParameterSetName = "Single")]
# Specifies the update Id we should import to WSUS
[ValidateNotNullOrEmpty()]
[String]$UpdateId,
[Parameter(Mandatory = $true, HelpMessage = "Specifies path to a text file containing a list of update ID's on each line", ParameterSetName = "Multiple")]
# Specifies path to a text file containing a list of update ID's on each line.
[ValidateNotNullOrEmpty()]
[String]$UpdateIdFilePath
)
Set-StrictMode -Version Latest
# set server options
$serverOptions = "Get-WsusServer"
if ($psBoundParameters.containsKey('WsusServer')) { $serverOptions += " -Name $WsusServer -PortNumber $PortNumber" }
if ($UseSsl) { $serverOptions += " -UseSsl" }
# empty updateID list
$updateList = @()
# get update id's
if ($UpdateIdFilePath) {
if (Test-Path $UpdateIdFilePath) {
foreach ($id in (Get-Content $UpdateIdFilePath)) {
$updateList += $id.Trim()
}
}
else {
Write-Error "[$UpdateIdFilePath]: File not found"
return
}
}
else {
$updateList = @($UpdateId)
}
# get WSUS server
Try {
Write-Host "Attempting WSUS Connection using $serverOptions... " -NoNewline
$server = invoke-expression $serverOptions
Write-Host "Connection Successful"
}
Catch {
Write-Error $_
return
}
# empty file list
$FileList = @()
# call ImportUpdateFromCatalogSite on WSUS
foreach ($uid in $updateList) {
Try {
Write-Host "Attempting WSUS update import for Update ID: $uid... " -NoNewline
$server.ImportUpdateFromCatalogSite($uid, $FileList)
Write-Host "Import Successful"
}
Catch {
Write-Error "Failed. $_"
}
}
After saving and running the PowerShell script, I received the error below.
If you notice below, the error is an exception stack trace, not trying to read data, but an exception calling WriteErrorException.
Diagnosing the Issue
When you encounter the “wsus import the underlying connection was closed” error, an accompanying error message usually includes an inner exception stack trace. This trace gives you valuable clues as to what exactly is causing the problem.
I have seen the forcibly closed error in the past troubleshooting other issues or connectivity from PowerShell and have found previously it relates to the encryption level.
The error often occurs during a sync attempt between the WSUS server and Microsoft Update Catalog. The existing connection can be forcibly closed due to an unexpected error, which leads to a sync failure.
The error message and the exception stack trace may reference registry keys, a Powershell script, or a specific file name in the Program Files folder.
Take note of anything out of the ordinary that may result in being better able to pinpoint the underlying reason for the connectivity or “closed” error.
The Fix: Change SchUseStrongCrypto registry key
It surprised me, but even in Windows Server 2022 with the latest patches, I saw the error message described in the post when using the new PowerShell import updates process.
The fix is relatively simple. You can use PowerShell to change the properties on a couple of registry keys related to .NET Framework and TLS settings.
Set-ItemProperty -Path 'HKLM:SOFTWAREWow6432NodeMicrosoft.NetFrameworkv4.0.30319' -Name 'SchUseStrongCrypto' -Value '1' -Type DWord
Set-ItemProperty -Path 'HKLM:SOFTWAREMicrosoft.NetFrameworkv4.0.30319' -Name 'SchUseStrongCrypto' -Value '1' -Type DWord
After running the above PowerShell commands on the WSUS server, reboot your WSUS server. You can do this with the following PowerShell cmdlet:
Restart-Computer
After I ran the commands and rebooted, I was able to run the PowerShell import command without any errors successfully:
ServerSyncProxy Proxy and WSUS Console
Another aspect that can lead to an “underlying connection was closed” error is the serversyncproxy proxy. Check its settings in the WSUS console, as incorrect configurations can cause the WSUS server to lose its connection to the internet or intranet.
Points for consideration
What is WSUS Server?
The acronym WSUS stands for Windows Server Update Services, a Microsoft product that enables administrators to manage Windows Udates released through Microsoft Update to computers and servers on a corporate network.
WSUS has played an integral part in the enterprise, maintaining system security and performance. However, it has been showing its age with the underlying technologies it uses to perform management, including Internet Explorer and ActiveX controls.
When you install WSUS it creates a WSUS administration website alongside the Default web site, allowing remote clients to connect.
The Role of TLS 1.2 and .NET Framework
The error might often be due to TLS 1.2 not being turned on. TLS 1.2, or Transport Layer Security, is like a security guard for online communication. It makes sure that the conversation between your apps and you on the internet is private and not meddled with by anyone else. So, when your computer (browser) talks to the servers (where websites and apps live), TLS 1.2 keeps that chat secure, ensuring no one else is listening or messing with your messages.
.NET framework helps programmers speak the same language, even when using different programming languages. It’s like a universal translator, allowing programmers to use multiple languages but still understand each other.
How Does WSUS Sync with Microsoft Update Catalog?
The WSUS server connects to the Microsoft Update Catalog to download updates. During this sync process, WSUS identifies the updates applicable to your infrastructure based on your configuration.
It’s crucial to keep this synchronization seamless, but sync failures like “wsus import the underlying connection was closed” can disrupt this process.
What Might Cause an Unexpected Error in WSUS?
Unexpected errors in WSUS can be attributed to many factors. One common issue is a mismatch in the TLS settings, with the WSUS server requiring TLS 1.2 for secure connections. Similarly, issues with the .NET Framework can cause unexpected errors.
It’s also essential to check the “webrequest request” and “serversyncproxy proxy” configurations in the WSUS console to ensure they’re not leading to an unexpected error.
Can Proxy Settings Impact WSUS Performance?
Yes, incorrect proxy settings in your WSUS server can impact its performance and lead to errors. Proxy settings are involved in managing connections between your WSUS server, its clients, and the Microsoft Update Catalog. Thus, any misconfiguration in the “serversyncproxy proxy” can cause connection-related issues.
Why Do WSUS Errors Often Involve Internet Explorer?
Even though Internet Explorer is considered outdated, WSUS still relies on it for certain operations. Issues can arise if Internet Explorer’s configuration isn’t compatible with the WSUS server’s requirements.
What is the Role of .NET Framework in WSUS Operations?
.NET Framework is a crucial component of the WSUS server. It provides the programming model for building and running WSUS and managing its connections.
Therefore, having an outdated or incompatible .NET Framework can cause issues, including connection problems.
Why Does an Existing Connection Get Forcibly Closed?
An existing connection might get forcibly closed due to a variety of reasons. It could be related to network issues, incorrect TLS settings, issues with the .NET Framework, or other system-level problems.
Can I Manually Import Updates in WSUS?
Manually importing updates into WSUS from the Microsoft Update Catalog is possible. Microsoft has introduced a new PowerShell Import method as the only supported way to import updates into your WSUS server.
Can WSUS Function Without Internet Explorer?
As of this writing, WSUS still relies on certain Internet Explorer settings for its operations. Therefore, completely eliminating Internet Explorer might result in WSUS issues.
Is There a Way to Access WSUS Server Through PowerShell?
Yes, using the “Get-WsusServer” command in PowerShell, you can access and manage your WSUS server. This can be helpful for troubleshooting and automating some management tasks.
Can the WSUS Error Log Provide Insight into the Problem?
Yes, WSUS maintains a log of its operations and is often a great resource when troubleshooting issues. If you’re seeing errors like “wsus import the underlying connection was closed,” you can often find more details about what happened in the log.
Final Thoughts and Further Troubleshooting
I suspect more will be running into the error message with the WSUS import updates process using the new PowerShell method outlined by Microsoft. It is surprising to see that the newest Microsoft OS’es, like Windows Server 2022, have issues with TLS versions and need to update registry keys. However, this seems to be the case in my Windows Server 2022 and WSUS testing. Let me know in the comments if you guys see anything different in your testing.
We had the same problem on Windows Server 2019 and your fix worked.
Thank you for your Post, saved us much time.
philbert,
Thank you for the comment and so glad the fix worked!
Brandon
Thank you so much, for your time and effort! Here, we are use Windows 2016 Server. Same problem. Solution worked! Greetings!
Paulo,
So glad this was helpful!
Brandon