PSWindowsupdate: Automated Windows Updates with PowerShell
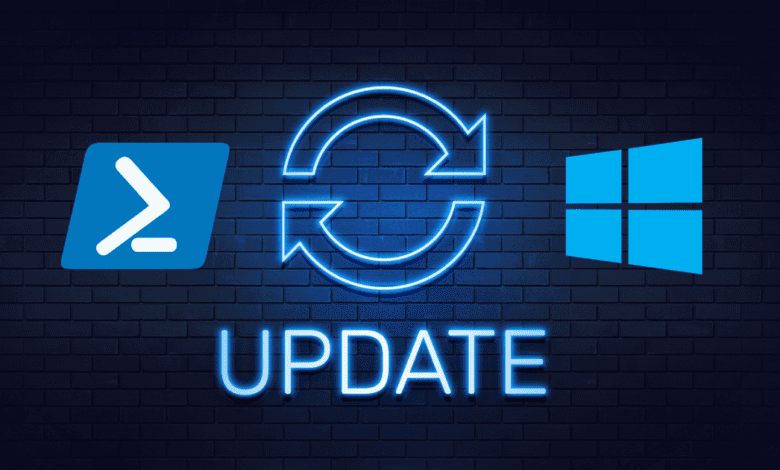
What is PSWindowsUpdate?
Admins can control every aspect of Windows updates with this module. These types of tasks include viewing available updates and initiating installations to setting update schedules. It includes advanced features like installing specific updates, managing hidden updates, or even automating the entire update process using the Windows Task Scheduler.
Installing the PSWindowsUpdate Module
The installation process for the PSWindowsUpdate module starts with the command line in your PowerShell console. You need to install the module from the PowerShell gallery by typing:
Install-Module -Name PSWindowsUpdate
This command will download and install the module on your local system. However, it’s critical to note that you may need administrator privileges to install modules. Open the PowerShell prompt as an admin and run the command.
You will be prompted to trust the untrusted repository, which is normal.
Type “Y” to trust the PSGallery repository.
Importing the PSWindowsUpdate Module
Once installed, you need to import the module PSWindowsUpdate to begin using it. Use the following command:
Import-Module -Name PSWindowsUpdate
It loads the module into your active PowerShell session, making the related cmdlets available for use.
Checking for Available Updates
Upon successful import of the PSWindowsUpdate module, you can quickly check for available updates using the command:
Get-WindowsUpdate
This command will query your machine’s Windows Update Client settings and connect to the Microsoft Update servers to fetch the list of all available updates. You can see critical updates, security updates, and all other types of updates that your system can download and install.
Downloading Windows Updates
The PSWindowsUpdate module offers the command:
Download-WindowsUpdate
Use this to download Windows updates. Depending on your settings, this command initiates the download process for all approved updates from the Windows Server Update Service (WSUS) or Microsoft Update.
Installing Windows Updates
With the updates downloaded, you can install them using the ‘Install-WindowsUpdate‘ command. This command installs all downloaded updates, following which your system might need to reboot.
Install-WindowsUpdate
Managing Windows Update History
The PSWindowsUpdate module provides an opportunity to access your system’s update history. You can use the ‘Get-WUHistory‘ command to get a detailed log of all installed updates.
Get-WUHistory
This command returns a detailed list, including the KB number, update title, and the status of the installed updates.
Installing Specific Updates
You may need to install a specific update related to a specific KB that has been released. We can do that with the PSWindowsUpdate module also. Note the following example of installing a specific update:
Install-WindowsUpdate -KBArticleID KB4012606 -AcceptAll -AutoReboot
This command will only install the specified update and perform an automatic reboot if necessary.
Installing Only Security Updates
If you wish only to install security updates, you can do so with the following command:
Get-WindowsUpdate -Category 'SecurityUpdates' | Install-WindowsUpdate
This command first fetches only the security updates and then pipes them into the Install-WindowsUpdate command, installing only the security updates.
Hiding Updates
There may be certain Windows Updates that you want to hide to keep these from being installed in your environment on machines. If you want to hide Windows updates, there is a specific cmdlet that you can use to do that called the Hide-WindowsUpdate command. If you want to hide the update with the KB number KB4012606, you can do that with the following command:
Hide-WindowsUpdate -KBArticleID KB4012606
This command will hide the specified update, preventing it from appearing in future searches for updates.
Checking if a Reboot is Required
You may want to see if there is a pending reboot required. If you want to check if a reboot is required after installing updates, you can do so with the Get-WURebootStatus command like so:
Get-WURebootStatus
This command will check and let you know if any installed updates require a reboot.
In the example, replace the placeholder KB numbers in the commands with the actual KB number of the update you’re interested in. Also, always ensure to run these commands in a safe and controlled environment, particularly when executing them on production systems or remote computers.
Automate Windows Updates using PSWindowsUpdate
How can you automate running PSWindowsUpdate scripts? Note the following code example:
# Import the PSWindowsUpdate module
Import-Module PSWindowsUpdate
# Get all available updates
$updates = Get-WindowsUpdate -MicrosoftUpdate
# Filter out optional updates
$importantUpdates = $updates | Where-Object {$_.IsDownloaded -eq $true -and $_.IsMandatory -eq $true}
# Install important updates
$importantUpdates | Install-WindowsUpdate -AcceptAll -AutoReboot
In this script, we first import the PSWindowsUpdate module. We then get all available updates using Get-WindowsUpdate. Using Where-Object, we filter out only the important updates, ignoring the optional ones. Then we can install the important updates using Install-WindowsUpdate, automatically accepting EULAs and rebooting if necessary.
We can automate it using Task Scheduler. Here’s a basic example of how you can do this:
Open Task Scheduler and create a new task.
In the Triggers tab, set the schedule for the task according to your needs (for example, daily at 3 AM).
In the Actions tab, select ‘Start a program’ and input powershell.exe as the program.
In the ‘Add arguments’ field, input -ExecutionPolicy Bypass -File “
c:\your script file path.ps1
“ where <your script file path> is the path to your PowerShell script.Finish the wizard and the task will be scheduled.
This script and scheduling are basic examples. You may need to modify the script and task parameters according to your specific requirements, such as filtering updates based on criteria or sending a report by email after installation.
Also, be sure to test these scripts in a safe and controlled environment before deploying them in production, especially when executing them on remote computers.
Using Automation in the home lab
Wrapping up
The PSWindowsUpdate module is a great way for system administrators to manage single or multiple Windows servers using a fully automated solution built on top of PowerShell. It has many capabilities that give you full control over the Windows Update service.